경험 많은 프로그래머의 시선으로 본 LLM과의 협업
LLM(대규모 언어 모델)의 급속한 발전은 때때로 벅차게 느껴집니다. 그래서 저는 간단한 Project Todo 를 하나 만들어보려 합니다. 오래전부터 이 분야에 몸담아온 저 같은 노년의 프로그래머에게는 특히 힘든게 아닌가 생각합니다.
처음엔 약간의 절망감도 들었습니다.
요즘 사람들이 코딩하는 방식—감각적인 프롬프트, 자동화 도구, AI 보조 워크플로우—이 모두가 낯설고 익숙하지 않았기 때문입니다.
하지만 직접 실험을 해보니, 작은 희망의 불씨가 피어났습니다.
아직 프롬프트 중심 개발에 능숙하다고는 할 수 없지만, 오랜 경험이 저에게 또 다른 무기를 안겨주었죠. 바로 큰 그림을 보는 능력입니다.
시스템을 어떻게 구조화할지 알고 있고, 문제를 작은 블록처럼 나누고 하나씩 조립해 나가면 결과가 훨씬 더 견고하고 신뢰할 수 있다는 걸 몸으로 배워왔습니다.
또한 저는 아키텍처 설계, 디버깅, 테스트에 대한 감각이 뛰어납니다. 이런 능력은 도구가 아무리 변해도 쉽게 바뀌지 않죠.
그래서 저는 이런 강점이 실제로 동작하는 실전용 소프트웨어를 만드는 데 있어 제게 경쟁력이 된다고 믿습니다.
이제는 LLM을 거부하는 대신 받아들이기로 했습니다. 단순히 코드를 생성하는 도구가 아니라, 함께 만들어가는 협업 파트너로요. 물론 아직 어색한 부분도 있습니다.
불과 얼마 전까지만 해도 저는 머신러닝 시스템을 ‘만드는’ 입장이었지, 그것과 ‘협업’할 거라고는 상상도 못했으니까요.
하지만 이 변화가 꽤 재미있을 수도 있겠다는 생각이 듭니다.
그리고 재미야말로 최고의 동기부여가 아닐까요.
LLM과의 대화 — 작은 장난감 프로젝트, Project Todo 만들기
휴대폰에서 쓸 수 있는 간단한 ToDo 앱을 만들고 싶어요. 어떻게 시작하면 될까요?
어떤 방식으로 만들지 결정하기
웹 앱으로 만들기?
→ HTML + JS (PWA로 만들면 실제 앱처럼 보일 수 있음)모바일 앱으로 만들기?
→ Flutter, React Native, Kotlin, Swift 등…
제 개발 환경이 좋진 않아요. 어떤 방식이 더 간단할까요?
→ 환경이 가볍다면 웹 앱 (PWA) 이 더 쉬워요.
왜?
설치할 필요 없이 브라우저만 있으면 되니까요.…
좋아요, 그럼 웹 방식으로 갈게요. 뭘 먼저 하면 되나요?
index.html
style.css
script.js
이제 각각의 기본 코드를 보여드릴게요:
index.html – V1
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Simple Todo</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>📝 Todo List</h1>
<input type="text" id="todo-input" placeholder="Input Todo..." />
<button id="add-btn">Add</button>
<ul id="todo-list"></ul>
<script src="script.js"></script>
</body>
</html>
style.css – V1
body {
font-family: sans-serif;
text-align: center;
margin-top: 50px;
}
input {
padding: 10px;
width: 200px;
}
button {
padding: 10px;
}
li {
margin: 10px 0;
}
script.js – V1
const input = document.getElementById('todo-input');
const addBtn = document.getElementById('add-btn');
const list = document.getElementById('todo-list');
addBtn.addEventListener('click', () => {
const text = input.value.trim();
if (text === '') return;
const li = document.createElement('li');
li.textContent = text;
li.addEventListener('click', () => {
li.style.textDecoration = li.style.textDecoration === 'line-through' ? '' : 'line-through';
});
list.appendChild(li);
input.value = '';
});
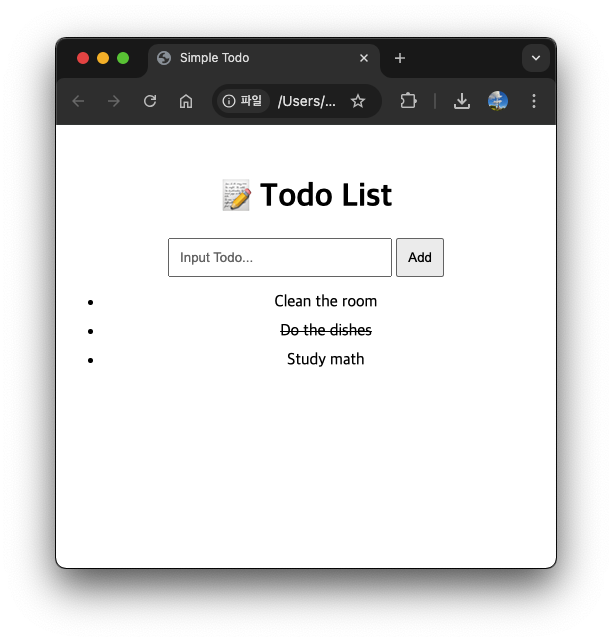
LocalStorage에 저장하고, 등록/완료 날짜를 자동으로 추가하고, 삭제 버튼도 만들 것.
추가된 시간 표시:
추가됨: YYYY-MM-DD HH:MM
❌ 버튼으로 삭제 가능
완료 시
completedAt
에 시간 저장완료된 항목은 취소선과 함께
(완료: 날짜)
표시다시 클릭하면 완료 취소되고
completedAt
초기화됨
Script.js – V2
const input = document.getElementById('todo-input');
const addBtn = document.getElementById('add-btn');
const list = document.getElementById('todo-list');
let todos = JSON.parse(localStorage.getItem('todos')) || [];
renderTodos();
addBtn.addEventListener('click', () => {
const text = input.value.trim();
if (text === '') return;
const newTodo = {
text,
done: false,
createdAt: new Date().toISOString(),
completedAt: null,
deletedAt: null
};
todos.push(newTodo);
saveTodos();
renderTodos();
input.value = '';
});
function renderTodos() {
list.innerHTML = '';
todos.forEach((todo, index) => {
if (todo.deletedAt) return;
const li = document.createElement('li');
const textSpan = document.createElement('span');
let displayText = todo.text;
if (todo.done && todo.completedAt) {
displayText += ' (Completed: ${formatDate(todo.completedAt)})';
} else {
displayText += ' (Add: ${formatDate(todo.createdAt)})';
}
textSpan.textContent = displayText;
if (todo.done) {
textSpan.style.textDecoration = 'line-through';
}
textSpan.addEventListener('click', () => {
todos[index].done = !todos[index].done;
todos[index].completedAt = todos[index].done ? new Date().toISOString() : null;
saveTodos();
renderTodos();
});
const deleteBtn = document.createElement('button');
deleteBtn.textContent = '❌';
deleteBtn.style.marginLeft = '10px';
deleteBtn.addEventListener('click', () => {
todos[index].deletedAt = new Date().toISOString();
saveTodos();
renderTodos();
});
li.appendChild(textSpan);
li.appendChild(deleteBtn);
list.appendChild(li);
});
}
function saveTodos() {
localStorage.setItem('todos', JSON.stringify(todos));
}
function formatDate(isoStr) {
const date = new Date(isoStr);
return date.toLocaleDateString() + ' ' + date.toLocaleTimeString();
}
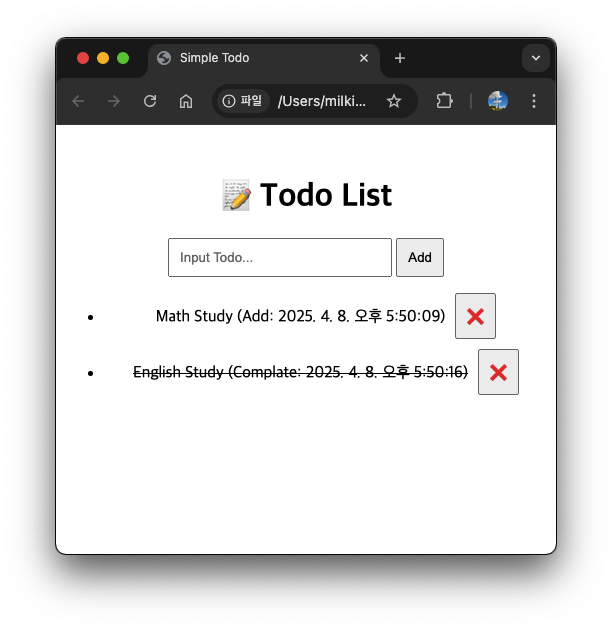
로컬에서 계속 테스트하고 있는데 사용 수 있는 무료 웹 서비스를 추천해줄 수 있나요?
1. Render (https://render.com)
- Use case: Full-stack apps (e.g., FastAPI + React)
- Free tier: Yes — free web services with auto-deploy from GitHub
- Local testing: Develop locally, then push to deploy
- Note: Free tier may sleep after inactivity
2. Vercel (https://vercel.com)
- Use case: Frontend frameworks (React, Next.js)
- Free tier: Yes — very developer-friendly
- Local testing:
vercel dev
command lets you test locally- Note: Best for frontend + serverless functions
3. Replit (https://replit.com)
- Use case: Quick prototyping, supports Python, JS, etc.
- Free tier: Yes — with instant preview in browser
- Local testing: Built-in, no setup required
- Note: Ideal for beginners or quick sharing
4. GitHub Codespaces (https://github.com/features/codespaces)
- Use case: Full VS Code in the browser
- Free tier: Yes, for small usage with GitHub Free
- Local testing: Dev container support, good for remote-like local testing
- Note: Needs GitHub + some setup
5. ngrok (https://ngrok.com)
- Use case: Expose your local server to the web
- Free tier: Yes — limited usage
- Local testing: Runs on your machine, makes it public
- Note: Great for testing webhooks, mobile devices
6. Glitch (https://glitch.com)
- Use case: Node.js and small web apps
지금은 동적 페이지를 동작시킬 필요는 없습니다.
1. GitHub Pages
- Best for: Hosting static websites from a GitHub repo
- How to use: Push your HTML/CSS to GitHub, enable GitHub Pages
- Free: 100% free
- URL example:
https://yourusername.github.io/yourproject
2. Netlify
- Best for: Static sites with build steps (e.g., HTML + Tailwind)
- Features: Drag-and-drop deploy, custom domain support
- Free: Yes, generous free tier
- Local testing: Use
netlify-cli
withnetlify dev
3. Vercel
- Best for: Static or frontend frameworks (e.g., Next.js, React)
- Deploy: Connect GitHub > Auto-deploy
- Free: Yes
- Local testing: Use
vercel dev
4. Cloudflare Pages
- Best for: Static site deployment via Git
- Free: Yes
- Fast CDN: Ultra-fast global edge hosting
- Bonus: No sleep downtime like some other free tier
Tell them how to upload to your Github page
Github – Page
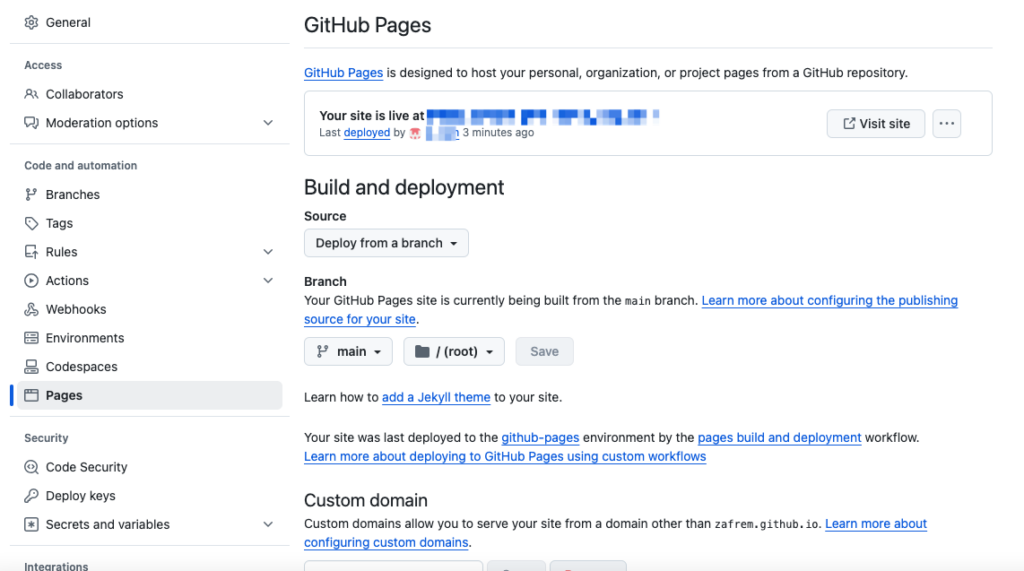
저는 오랫동안 깃허브를 사용해왔는데, 이제 이 Page 기능을 처음 사용해보고 있습니다. Project Todo 를 해보면서 처음 웹 프로그래밍을 접해서 좀 어렴움이 있었지만 나름 수월하게 프로그램이 개발되네요.
시스템 프로그래밍만 해봐서 꽤 생소했지만 재미있고 빠르기도 합니다.